As a new developer, the number of JavaScript libraries and frameworks available can be overwhelming.
How do you know which ones you should learn to use? Which will be most useful in your coding career? In this special guest post, Erik Hanchett gives us an overview of his top eleven JavaScript libraries and frameworks.
Here’s Erik!
—
In this post, we’ll be discussing 11 JavaScript libraries and frameworks and what they’re used for. I’ve tried to pick some of the most popular JavaScript libraries and frameworks, plus a few you may not have heard before.
This list of JavaScript libraries and frameworks is intended to serve as an introduction for beginners, so you can get an idea about what’s out there and why you should use these top JavaScript frameworks and libraries. When working as a web developer you have a lot of options, which makes it important to know which JS libraries and frameworks are best for which scenarios.
Table of Contents
- What Are JavaScript Libraries?
- What Are JavaScript Frameworks?
- How to Choose JavaScript Libraries and Frameworks
- JavaScript Libraries
- Javascript Frameworks
Disclosure: I’m a proud affiliate for some of the resources mentioned in this article. If you buy a product through my links on this page, I may get a small commission for referring you. Thanks.
What’s the Difference: JavaScript Libraries vs. Frameworks
A question I hear quite often is “What’s the difference between JavaScript libraries and frameworks?” That’s a good question, and there has been a lot of discussion on it online. Let’s quickly break down, what is a JavaScript library vs framework?
What Are JavaScript Libraries?
Generally, a JS library is a reusable piece of code that oftentimes has one primary use case. A library can consist of several functions/objects/methods, depending on the language. Your application can “link” to a library to allow you access to that functionality. JavaScript libraries prevent you from having to reinvent the wheel for certain snippets of code. This leads to faster, easier development with fewer errors.
There are around 83 JavaScript libraries available, covering machine learning (TensorFlow), rapid prototyping (Meteor), animated 3D graphics (Three.js), DOM manipulation (Dojo Toolkit), and more!
What Are JavaScript Frameworks?
On the other hand, a JS framework has more control of your app. It helps direct you on the architecture and the project that follows. JavaScript frameworks consist of multiple libraries and they provide you with hooks and callbacks, so you can continue to build upon them.
There are around 24 JavaScript frameworks out there, including testing frameworks (Mocha, Jasmine), JS frameworks for hybrid mobile apps (Ionic), frameworks for developing rich UIs (Webix), and more. The most popular JS frameworks are listed in this article, and include some top JavaScript frameworks you may have heard of like Angular, Vue, and Bootstrap.
JavaScript frameworks and libraries are both useful and it’s a good idea to experiment and see which ones work best for you. The main difference lies in who calls who (as explained in this video). In a framework, the framework code calls on your code, while in a library, it’s your code that makes calls to the library.
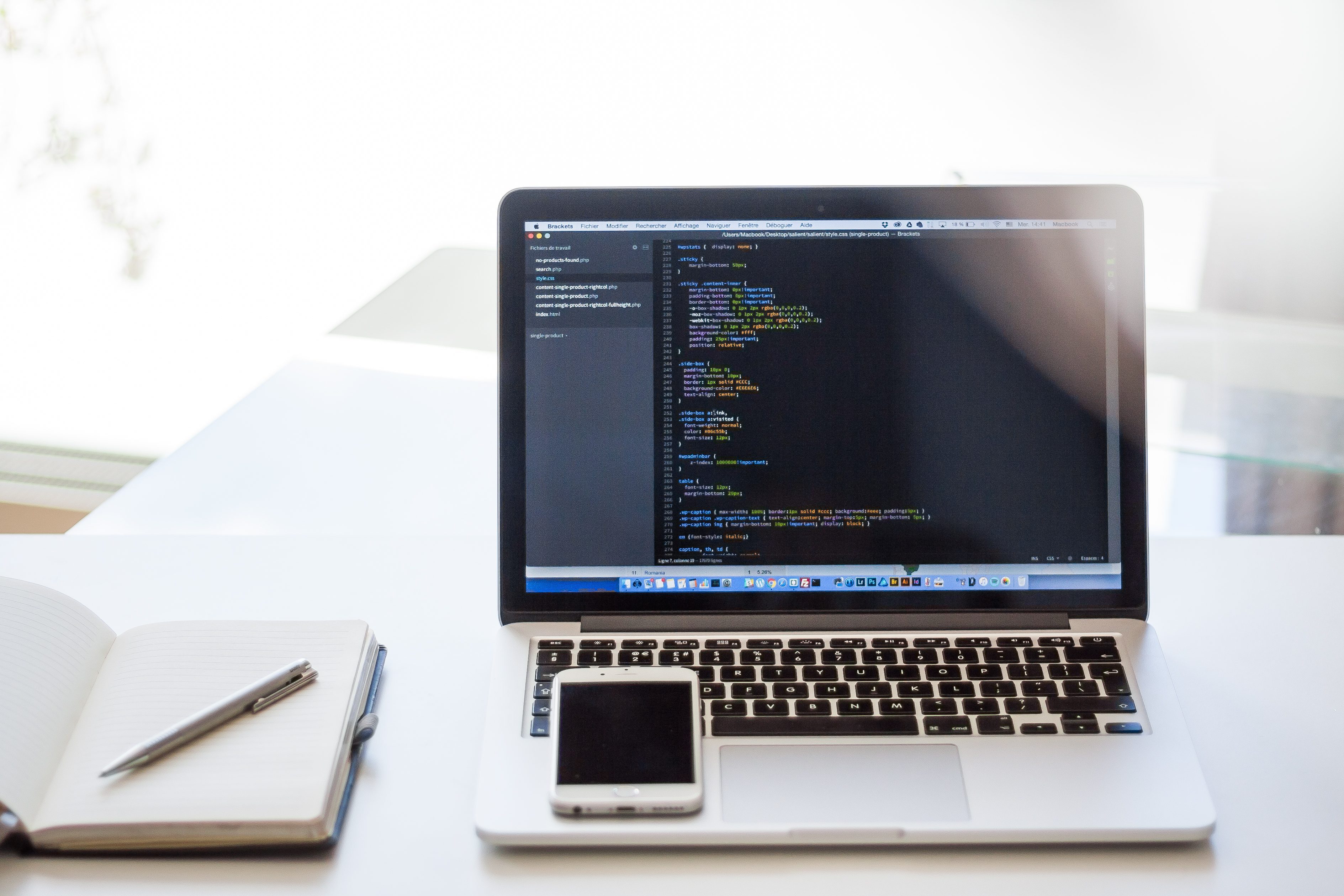
☝️ Back to the table of contents
How to Choose JavaScript Libraries and Frameworks
With so many options available (80+ libraries and 20+ frameworks), it can be overwhelming to choose the right ones for you. 🤯
One way to narrow down your decision is to look at job opportunities in your area. What does the demand look like? What are the most used JavaScript libraries and frameworks in the listings? For example, if there aren’t any job postings that require Underscore, you might want to go with more common JavaScript libraries like React.
Another way is to think about the goals of the project you’re working on. How big is your project, for example? That can help you narrow down your options and rule out libraries/frameworks that aren’t good for smaller projects, for instance.
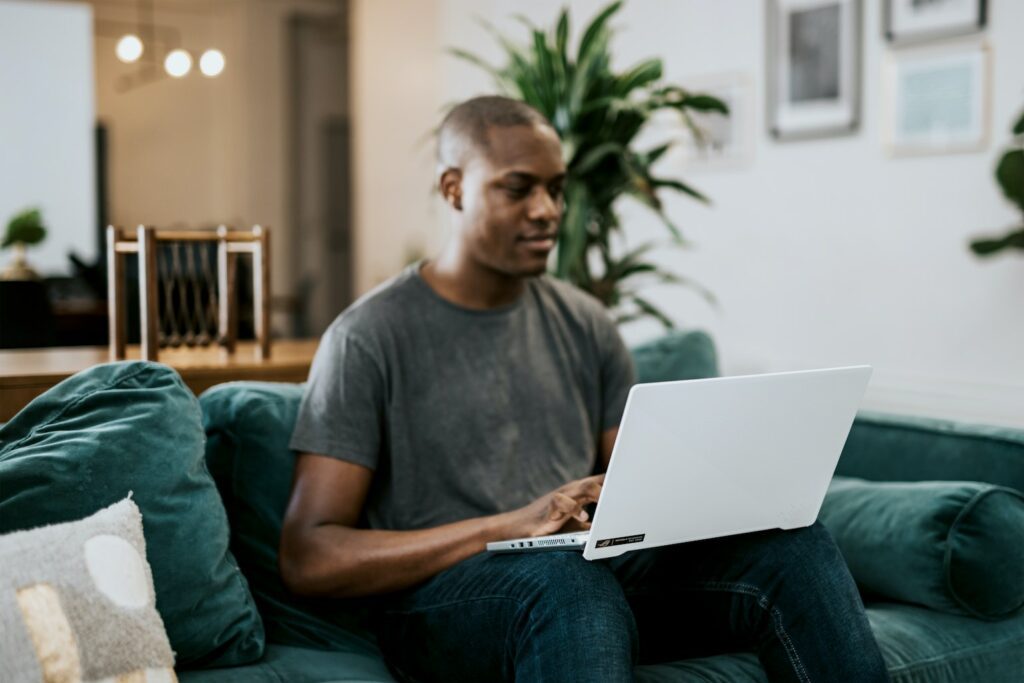
Yet another way is to see how big the community is and the number of learning resources/tutorials that are out there. This is especially important for beginners, as you don’t want to pick too small or new JavaScript libraries or frameworks that have poor documentation and a tiny community you can’t go to for help.
Below, I’ll go over all of this information about each JS library and framework so you can make an informed decision.
One thing to note is that having a solid foundation in JavaScript is key. When you have a good understanding of JS, you’ll start to see what you’re missing/what you need the most help with in your code and can pick a framework/library accordingly.
☝️ Back to the table of contents
TL;DR: JavaScript Libraries and Frameworks
JavaScript Library Summaries
JAVASCRIPT LIBRARY
SUMMARY
jQuery
- Very popular; half of all known websites use it
- Takes care of all cross-browser incompatibilities
- Useful to know; you will almost certainly run into older code bases that use jQuery
Underscore & Lodash
- Powerful functional programming helpers (e.g., map, filter and reduce)
- Helps you manipulate arrays, objects, and other functions
D3.js
- Great for creating custom data visualizations
- Powerful API that uses selectors
React
- Open-source library backed by Facebook
- Useful in creating interactive websites
Redux
- Most popular state management library
- Used most commonly with React
Backbone.js
- Simplifies your code
- Structure JS code in a MVC design
JavaScript Framework List
JAVASCRIPT FRAMEWORK
SUMMARY
Bootstrap
- Makes it easy to create responsive websites (even if you have limited design skills)
- Includes CSS styles, icons, components, & JavaScript plugins
Angular & AngularJS
- Front-end JS framework created & maintained by Google
- Can be inserted into HTML to give applications more functionality
Ember.js
- Features an excellent build tool called Ember CLI
- Offers everything you need to get started
Aurelia
- Very forward-thinking framework; uses a lot of newer ECMAScript features
- Powerful and composable
Vue.js
- Unlike other frameworks, you can easily mix and match Vue into projects
- Features include components, templates, transitions, & 2-way data binding
☝️ Back to the table of contents
List of JavaScript Libraries
Let’s start with libraries! 👇
Please note that pricing listed below may change in the future!
jQuery: An Old Classic (But Is It Obsolete?)
In the JavaScript world, jQuery is still, by far, one of the most prolific and popular JavaScript libraries out there. It was released in 2006 by John Resig and has been used in websites throughout the world. jQuery is used by 95.4% of all the websites whose JS library we know, which is 78.4% of all websites. That’s hundreds of millions (if not billions) of websites.
So what is jQuery? jQuery is a library used primarily for Document Object Model (DOM) manipulation. The DOM is a tree-like structure that represents all elements on a webpage.
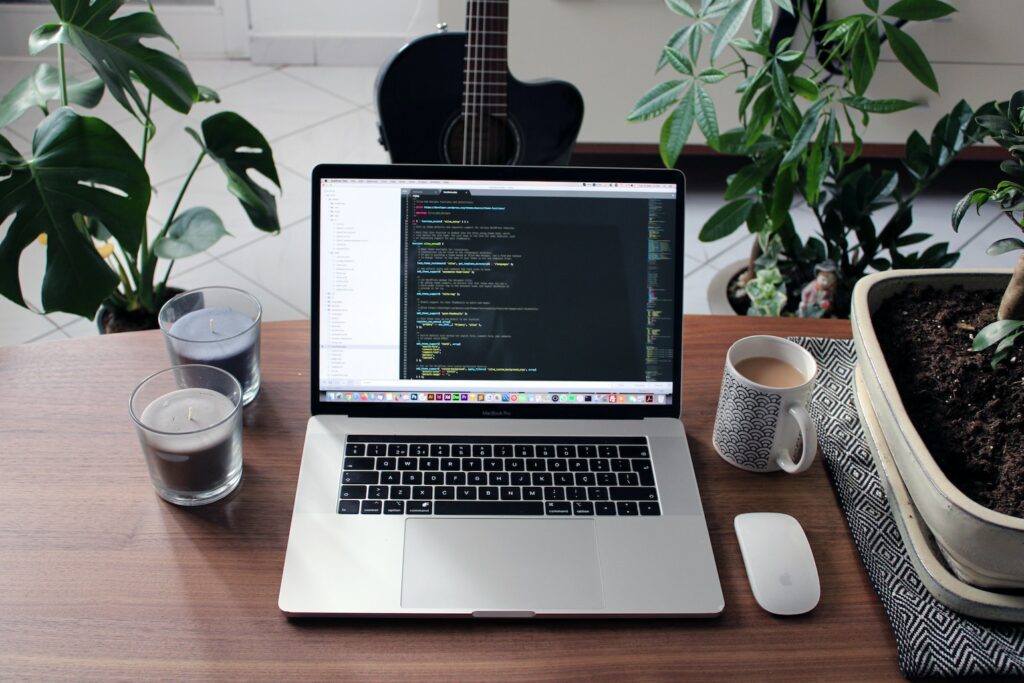
The jQuery library can select DOM elements, create animations, handle events, and more. Its goal is to be extensible, simple, and clear to use. It takes care of all cross-browser incompatibilities, and it promotes the separation of HTML and JavaScript.
Should you use jQuery? Well, it depends. Some people insist that jQuery is no longer needed. Modern browsers have greatly improved their DOM Application Programming Interfaces (API). In the past, you might have used jQuery to do simple query selections. You can now easily do that with plain old JavaScript.
Furthermore, with the popularity of single-page-application (SPA) frameworks it doesn’t make sense to use jQuery in many circumstances. For example, most SPAs have some sort of event handling built in. Using jQuery could cause more harm than good in these cases.
At the very least, you should learn the basics of jQuery as a new web developer. You will almost certainly run into older code bases that use jQuery, and it does come in handy in other scenarios from time to time.
➡️ See the jQuery JavaScript Library on GitHub
Pros & cons of jQuery
Pros
- Super easy to use; short learning curve
- Cross-browser compatible
- Highly flexible
Cons
- Can sometimes lead to code that is difficult to debug
- Slower than CSS
- You can do similar things in plain JS
💻 Where to learn it: Learn jQuery on Codecademy
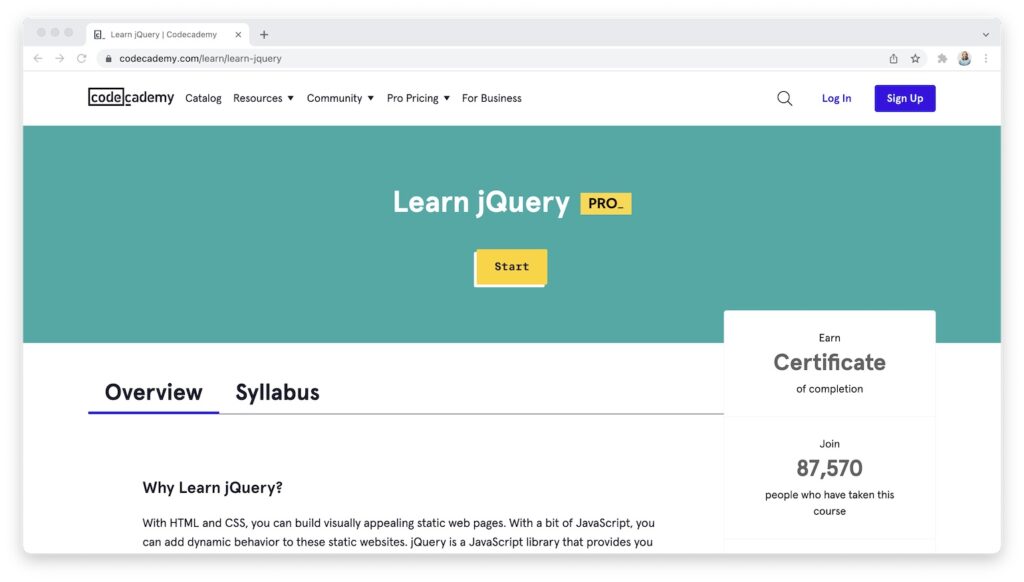
💡 What the course covers: The fundamentals of manipulating elements on a webpage and responding to user interactions—with jQuery.
⬇️ Course facts:
- Course Name: jQuery
- Platform: Codecademy
- Price: $19.99/month with a Codecademy subscription
📈 Skill level: Beginner
☝️ Back to the table of contents
Underscore and Lodash: Utility JS Libraries
In 2009, Jeremy Ashkenas created Underscore. Underscore is a utility library that consists of over 100 functions. These functions will help you manipulate arrays, objects, and other functions.
One of the most powerful features of Underscore is its functional programming helpers. For example, map, filter and reduce are some of the most popular. Functional programming (FP) is a programming paradigm that avoids changing state and mutating data. FP has become increasingly popular in the last few years, but it’s beyond the scope of this article today.
In 2012, Lodash had its first initial release. John-David Dalton created this library to have a more consistent cross-environment iteration support for arrays, strings, and argument objects.
In the process, Lodash has become a superset of Underscore with more features and better documentation. Today most developers have moved over to Lodash from Underscore. Even many of the contributors to Underscore now work on Lodash.
There is concern that Lodash is no longer needed as JavaScript has evolved. As the JavaScript standard evolves, new releases have included some of the utilities that you used to only be able to get from external libraries like Lodash and Underscore.
Nevertheless, Lodash and Underscore are excellent libraries that are especially helpful for any FP apps.
Pros & cons of Lodash and Underscore
Pros
- Makes your code more concise
- Cross-browser compatible
- Good documentation
Cons
- Problems aren’t always easy to spot
- You are adding an external dependency to your application
- Plain JS can sometimes be faster
💻 Where to learn it: Underscore.js Fundamentals on Pluralsight
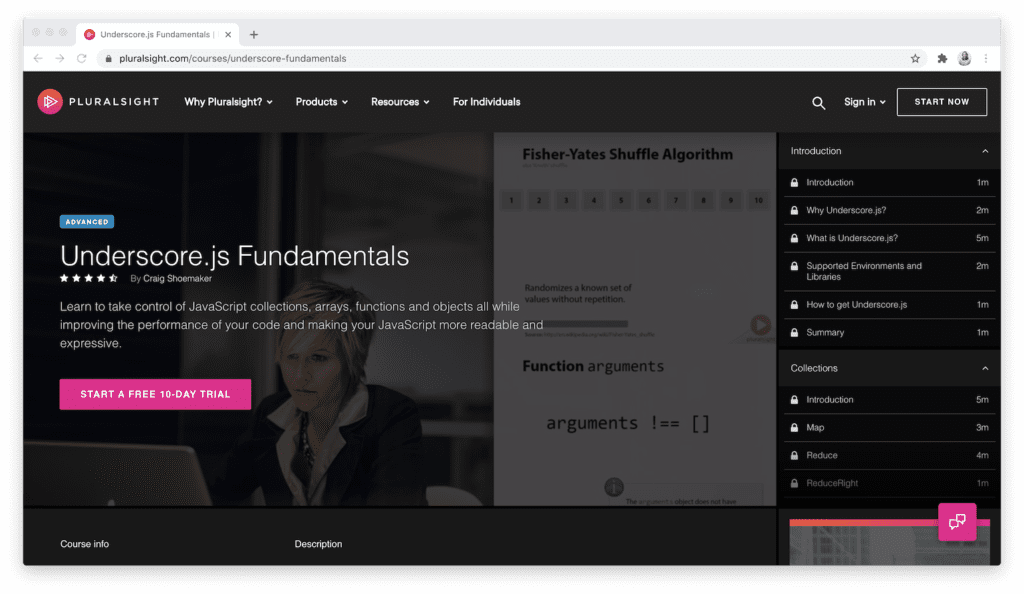
💡 What the course covers: How to manipulate and control JavaScript collections, arrays, objects and functions—with Underscore.js.
⬇️ Course facts:
- Course Name: Underscore.js Fundamentals
- Platform: Pluralsight
- Instructed by: Craig Shoemaker
- Price: $29/month with a Pluralsight subscription
📈 Skill level: Intermediate
☝️ Back to the table of contents
Start coding now
Stop waiting and start learning! Get my 10 tips on teaching yourself how to code.
D3.js: a JS Library for Graphing and Visualizations
D3.js is a JavaScript library for visualizing data. This library was developed in 2011 from a previous project called Protovis. Mike Bostock, Jeff Heer, and Vadim Ogievetsky developed D3.js to be more expressive than its predecessor and more compliant with current web standards.
D3.js is a great tool when creating custom visualizations. This could be something as simple as a bar chart, or as complex as a 3D surface plot. The library has a powerful API that uses selectors, much like the ones you would see in jQuery. After selecting an element in the DOM you can do all sorts of transitions and manipulations.
D3 7.2.0 the latest version of D3, is a collection of official and community-developed modules. Each has a different purpose. Some make it easy to work with colors; others work with dragging-and-dropping SVG elements.
If you’re looking to do any sort of visualization, this is the JS library to check out!
➡️ See the D3 JavaScript Library on GitHub
Pros & cons of D3.js
Pros
- Easy to customize; lots of ways to visualize data
- Huge community backing it
- Reusable components
Cons
- Steep initial learning curve
- Poor documentation
- Difficult for creative visualizations
💻 Where to learn it: Information Visualization: Programming with D3.js on Coursera
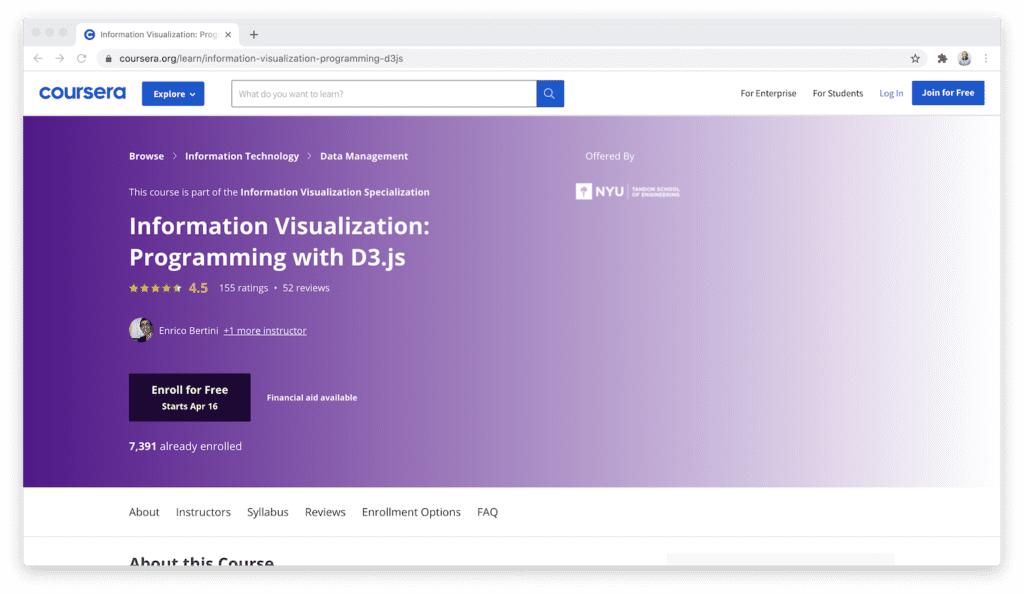
💡 What the course covers: The basics of creating visualizations with D3.js, including a fully working visualization system to visualize airline routes.
⬇️ Course facts:
- Course Name: Information Visualization: Programming with D3.js
- Platform: Coursera
- Instructed by: Enrico Bertini and Cristian Felix
- Price: $39/month with a Coursera subscription
📈 Skill level: Beginner
☝️ Back to the table of contents
React: Facebook’s Favorite Javascript Library
In March 2013, Jordan Walke released React while working at Facebook. Since then, React has become one of the most popular front-end libraries and is used in hundreds of thousands of websites around the world.
React (or React.js as it’s sometimes called) is an open-source library backed by Facebook. It’s used to help create small- or large-scale web applications and is particularly useful in creating interactive websites. Its tag line is “A JavaScript Library For Building User Interfaces”.
React is sometimes called a SPA, or single-page application. This term refers to web applications that can fit on a single web page and don’t require constant refreshes from the web browser. Content is loaded dynamically when needed using JavaScript.
It uses components, which help encapsulate code and state. Using components makes it easy to construct complicated user interfaces.
React uses JSX, which is an XML-like syntax that combines JavaScript and HTML. It’s not a templating language; it’s full-on JavaScript. Some new developers might find JSX a little confusing at first. However, after working with it for a while you’ll understand how beneficial it is. For example, JSX makes it easy to wrap JavaScript expressions directly inside your HTML.
React has a lot going for it, and it’s definitely a library you should look out for if you’re into front-end web development.
➡️ See the React JavaScript Library on GitHub
Pros
- Easy to learn and use
- Made up of reusable components
- Uses a virtual DOM to improve memory and efficiency
Cons
- Poor documentation
- JSX syntax can be difficult to grasp
- Can be hard to keep up changes
💻 Where to learn it: Mastering React on Code With Mosh
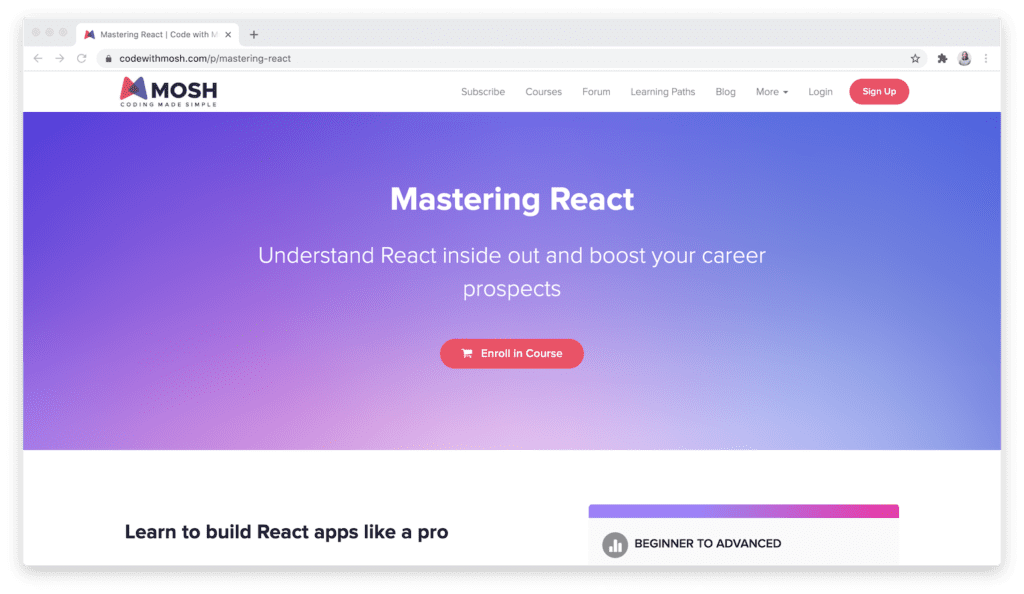
💡 What the course covers: How to build and deploy fast and interactive React apps with confidence, including React Native (for building mobile apps).
⬇️ Course facts:
- Course Name: Mastering React
- Platform: Code With Mosh
- Instructed by: Mosh Hamedani
- Price: $19 for single course or $29/month for all-access membership
📈 Skill level: Beginner
☝️ Back to the table of contents
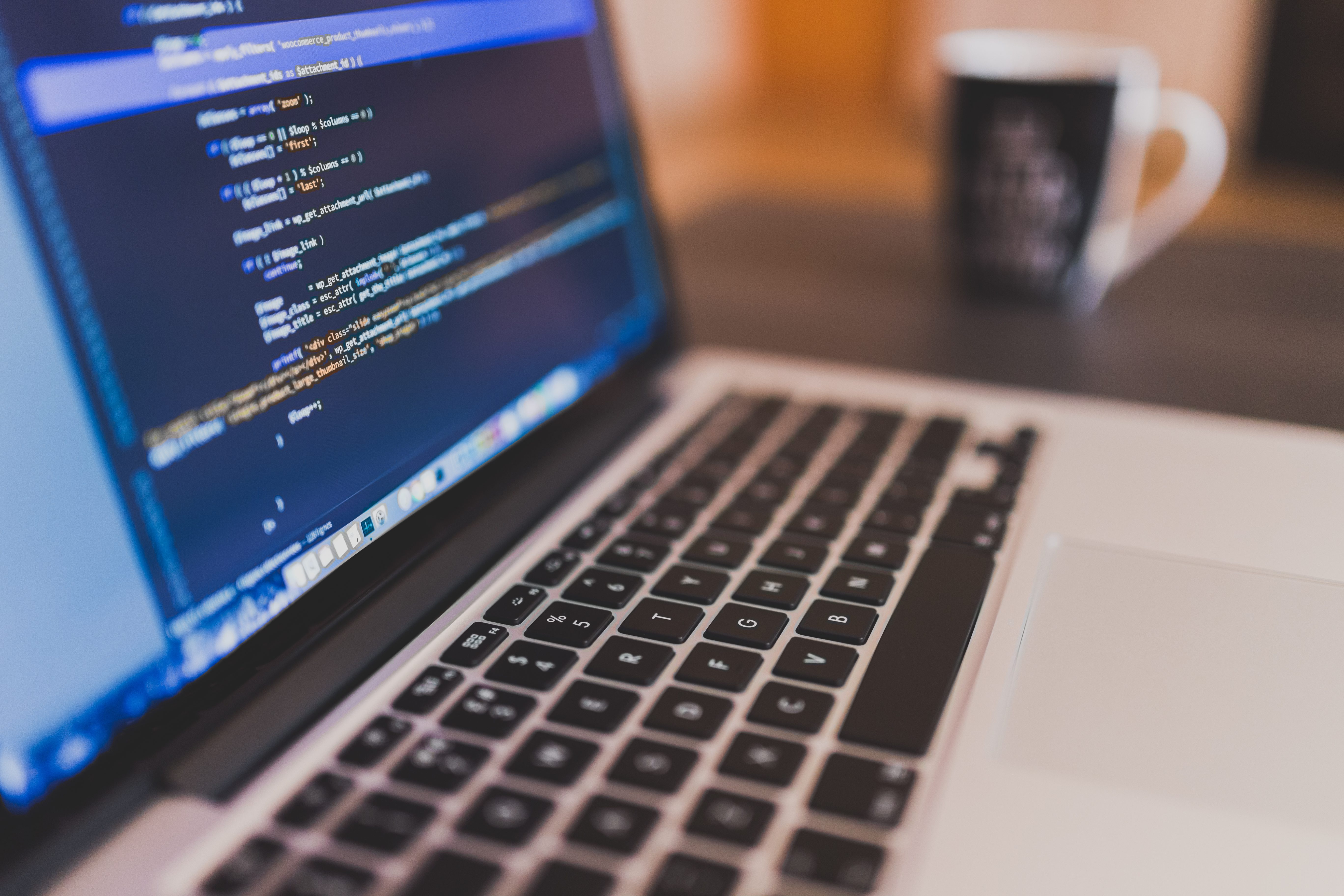
Redux: A Predictable State Container
Redux is the most popular state management library for JavaScript apps. State management is all about making the different components within apps (e.g., text fields, buttons) behave properly and react to each other.
Redux works by providing a central store that holds all states of your application. Each component can access the stored state without having to send it from one component to another.
It is used most commonly with React, but can also be used with Angular, Vue, or vanilla JavaScript for building user interfaces. The library was created by Dan Abramov and Andrew Clark in 2015.
➡️ See the Redux JavaScript Library on GitHub
Pros
- Easy; similar to writing HTML
- Debugging and testing is easy
- Every actions produces a predictable outcome
Cons
- Restricted design
- Possible security issues since any component can access the data
- High memory usage
💻 Where to learn it: The Ultimate Redux Course on Code With Mosh
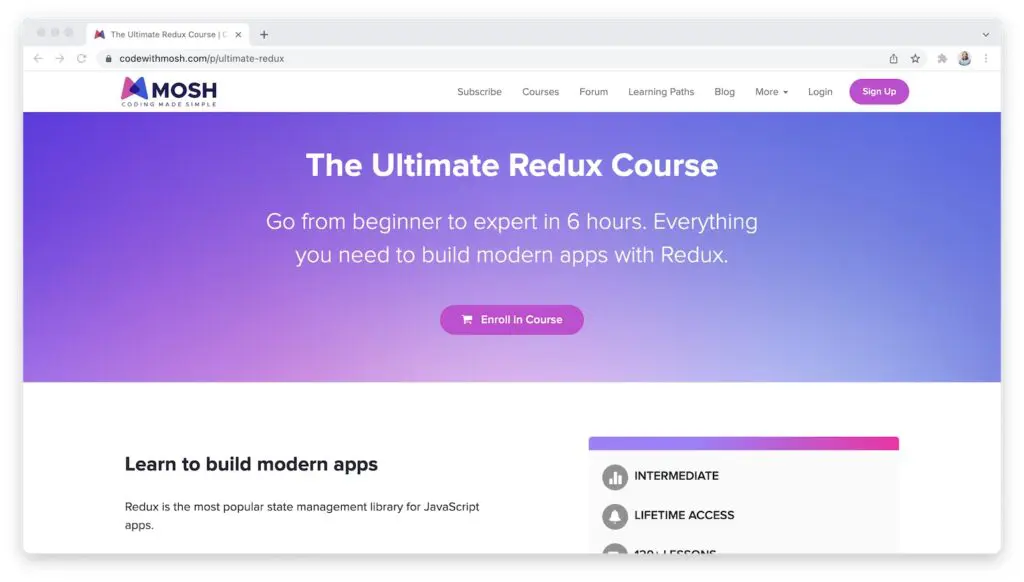
💡 What the course covers: How to use Redux Toolkit to kickstart Redux apps, write clean, concise, and maintainable Redux code, and more.
⬇️ Course facts:
- Course Name: The Ultimate Redux Course
- Platform: Code With Mosh
- Instructed by: Mosh Hamedani
- Price: $15 for single course or $29/month for all-access membership
📈 Skill level: Intermediate
☝️ Back to the table of contents
Backbone.js: Giving Structure to Web Applications
Backbone.js allows you to structure your JS code in a MVC (Model, View, Controller) application design paradigm. It helps keep your client-side JavaScript clean through the most common MVC patterns. Backbone is used to create single page web applications where only a single HTML page is sent to the browser, and every other interaction after that is handled by AJAX requests and JavaScript logic.
Backbone.js was created in 2010 by Jeremy Ashkenas, who is also known for CoffeeScript and Underscore.js. The goal of Backbone is to simplify your code. It provides a well organized and structured way to develop applications.
➡️ See the Backbone.js JavaScript Library on GitHub
Pros & cons of Backbone.js
Pros
- Fast and lightweight
- Super flexible
- Huge community
Cons
- Takes a longer development time
- Need deep understanding of inner workings
- Hard to unit test Backbone Views
💻 Where to learn it: Backbone.js Breakdown #1: Novice to Knowledgeable on Skillshare
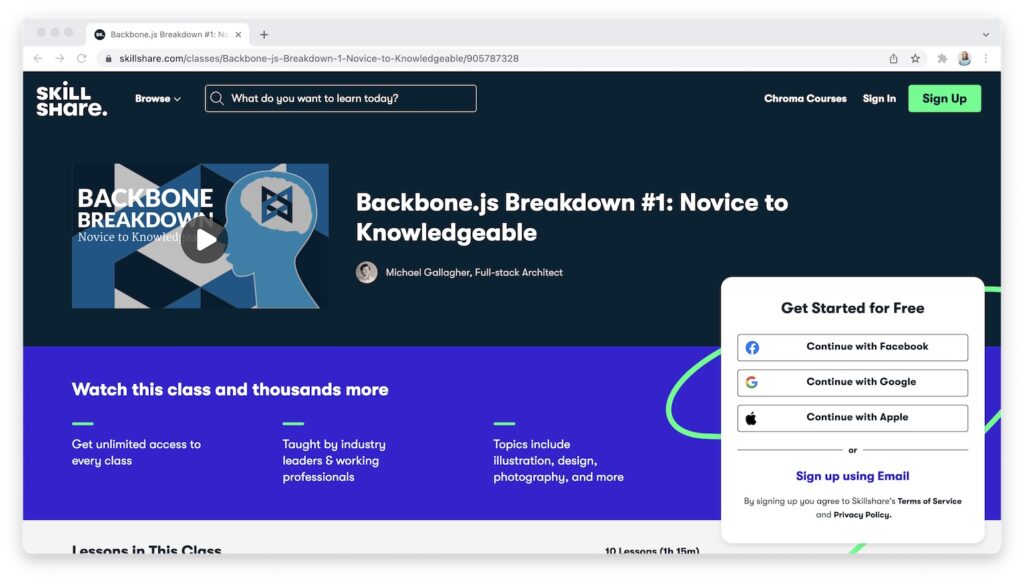
💡 What the course covers: The lifecycle of components within Backbone.js, including a deep dive into Backbone Events and a look under the hood of Collections and Models.
⬇️ Course facts:
- Course Name: Backbone.js Breakdown #1: Novice to Knowledgeable
- Platform: Skillshare
- Instructed by: Michael Gallagher
- Price: $15/month (paid annually) with a Skillshare subscription
📈 Skill level: Intermediate (requires knowledge of HTML and vanilla JavaScript)
☝️ Back to the table of contents
List of Javascript Frameworks
Now we’ll take a look at some of the top JavaScript frameworks.
Bootstrap: JavaScript Framework for Those Who Dislike Design
Bootstrap is an open-source front-end framework to help you with designing websites. On the Bootstrap website, the tagline is “Bootstrap is the most popular HTML, CSS, and JS framework for developing responsive, mobile-first projects on the web”.
It was originally named Twitter Blueprint and was developed by Mark Otto and Jacob Thornton while they worked at Twitter. Its first initial release was in 2011.
Bootstrap’s greatest strength is how easy it is for non-designers to quickly create decent-looking websites. Not to mention, Bootstrap makes it easy to create responsive designs (websites that adjust dynamically according to the device used: tablets, mobile phones, etc).
It features a number of CSS styles, icons, components, and JavaScript plugins. These plugins include a lot of common things you might use when building a website. This includes modals, dropdowns, alerts, and buttons, to name a few.
There has been some criticism of Bootstrap. One criticism I see a lot is that because of its popularity, there are a lot of websites built on Bootstrap that look very much alike, making it harder to build a unique-looking website. Keep that in mind.
If you’re starting to learn web development but aren’t into the design aspect, check out Bootstrap.
➡️ See the Bootstrap JavaScript Framework on GitHub
Pros & cons of Bootstrap
Pros
- Easy to use
- Encourages consistency in code
- Helps create responsive, mobile-friendly websites
Cons
- All Bootstrap websites look similar; can be too recognizable
- Can lead to slower loading times
- Not always easy to make customizations
💻 Where to learn it: Bootstrap 4 From Scratch With 5 Projects on Udemy
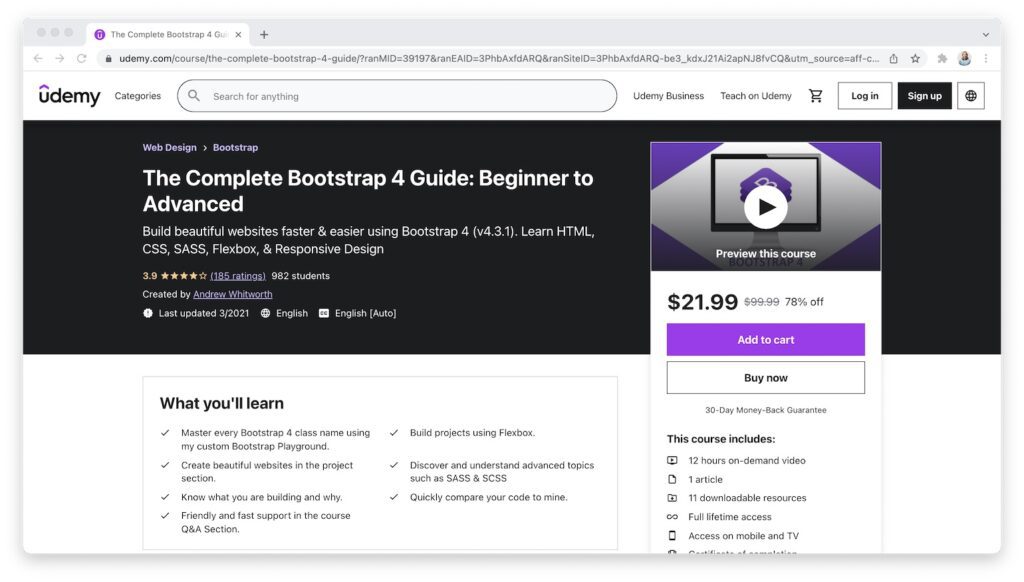
💡 What the course covers: How to use Bootstrap to implement mobile first web pages with CSS and JavaScript.
⬇️ Course facts:
- Course Name: Bootstrap 4 From Scratch With 5 Projects
- Platform: Udemy
- Instructed by: Brad Traversy
- Price: $84.99 (but Udemy often has sales)
📈 Skill level: Intermediate
☝️ Back to the table of contents
Angular and AngularJS: Google’s JavaScript Framework
AngularJS, also known as AngularJS 1.x, is an open-source JavaScript framework created and maintained by Google. Its initial release was in 2010.
AngularJS is a front-end framework that helps you create web applications. It’s also a SPA framework similar to React or Ember.js.
AngularJS uses directives that can be inserted into the HTML to give the application more functionality. It also supports two-way data binding.
Angular, sometimes known as Angular 2+, is a TypeScript-based open-source front-end framework. It’s a complete rewrite of AngularJS. It’s more modular, it recommends the use of TypeScript, and a simpler expression syntax.
At first, a lot of people were worried that Google would abandon Angular 1 when Angular 2+ came about. Fortunately, it seems that both projects are being maintained.
So what should you start with, Angular 1 or Angular 2+? I would recommend starting with 1, since there are a lot more jobs out there for 1 right now. In general, Angular is a great framework to look into if you’re a new dev.
➡️ See the Angular JavaScript Framework on GitHub
➡️ See the AngularJS JavaScript Framework on GitHub
Pros & cons of Angular and AngularJS
Pros
- Improves server performance
- Easy to test
- Good for quick prototyping
Cons
- Only for the front-end so you’ll need a way to organize your back-end code
- Can become unnecessarily complex
- Poor documentation
💻 Where to learn it: Learn AngularJS 1.X on Codecademy
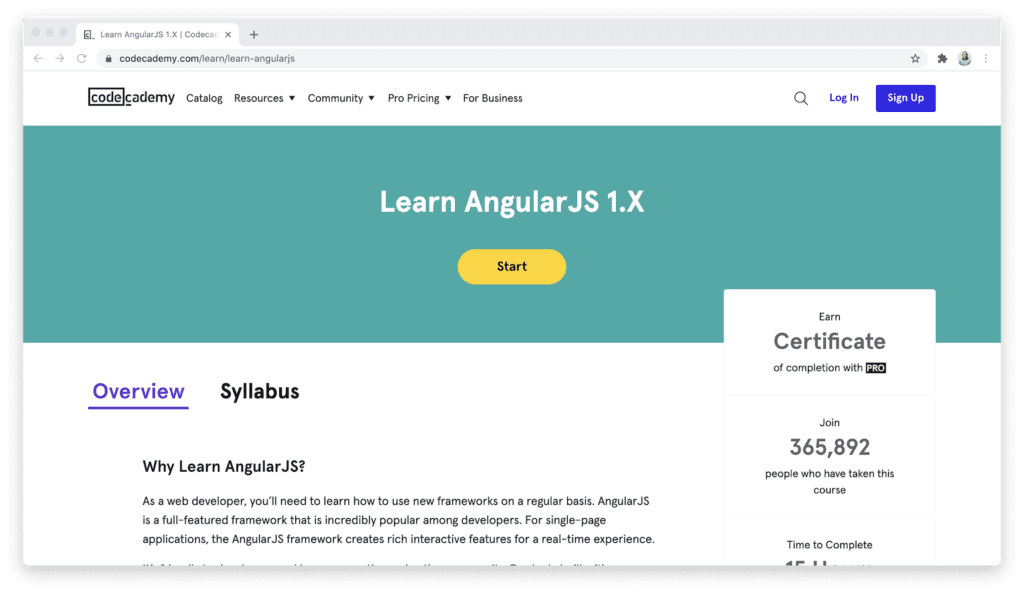
💡 What the course covers: Apply your understanding of HTML and JavaScript to build single-page web applications with AngularJS. You’ll be introduced to the Model-View-Controller (MVC) programming pattern and get a chance to build your own application from scratch.
⬇️ Course facts:
- Course Name: Learn AngularJS 1.X
- Platform: Codecademy
- Instructed by: Several instructors
- Price: $19.99/month with a Codecademy Pro subscription
📈 Skill level: Intermediate (strong foundation of JavaScript and basic knowledge of HTML required)
☝️ Back to the table of contents
Ember.js: the Batteries-included JavaScript Framework
Ember.js is an open-source JavaScript framework that was initially released in 2011 by Yehuda Katz. It was initially known as SproutCore 2.0 before it came to be known as Ember.js.
It’s no secret that Ember.js is one of my favorite JavaScript frameworks. I even wrote a book on it! The main thing I liked about Ember is its “batteries included” mentality. Ember features an excellent build tool that many other SPA frameworks have borrowed, called Ember CLI.
This build tool offers everything you need to get started. Need a router? It’s built in. Need testing? That’s built in too. Need to work with data from a back-end? There is Ember Data.
Ember.js follows many of the same principals that Ruby on Rails has. It’s highly opinionated, flexible, and prefers convention over configuration.
Ember.js is just getting better, and I’d highly recommend it.
➡️ See the Ember JavaScript Framework on GitHub
Pros & cons of Ember.js
Pros
- Has everything you need to get started
- Super flexible
- Strong ecosystem
Cons
- Harder to learn than other JS frameworks
- Heavy and highly opinionated
- Not suitable for smaller projects
💻 Where to learn it: Ember.js Essential Training on LinkedIn Learning
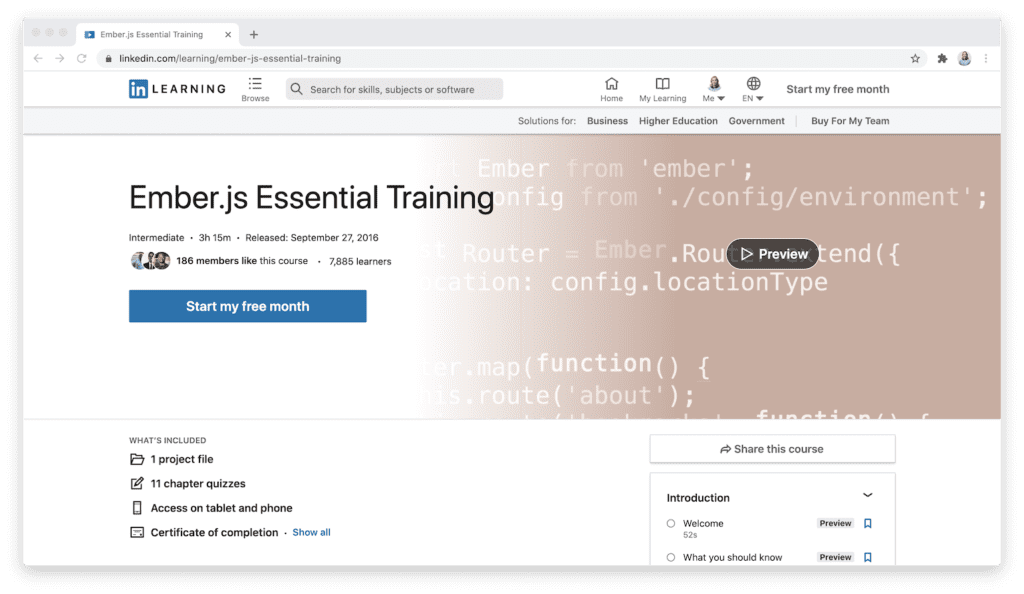
💡 What the course covers: Intro to Ember’s routers and models, as well as how to build a new project from scratch, using templates to create simple pages and dynamically load content with components and helpers.
⬇️ Course facts:
- Course Name: Ember.js Essential Training
- Platform: LinkedIn Learning
- Instructed by: Justin Yost
- Price: $26.99/month (paid annually) with a LinkedIn Learning subscription
📈 Skill level: Intermediate
☝️ Back to the table of contents
Aurelia: the Forward-looking JavaScript Framework
Aurelia is a front-end, open-source framework created by Rob Eisenberg. Aurelia 1.0 was first released in 2016.
Aurelia’s tagline is “A JavaScript framework for mobile, desktop, and web, leveraging simple conventions and empowering creativity”.
Aurelia is another SPA like React, Angular, and Ember.js. It’s powerful and composable. You can use one or all of its different modules to create your application. It offers routing, two-way data binding as well.
Aurelia is a very forward-thinking framework. It uses a lot of newer ECMAScript features (ECMAScript helps dictate JavaScript standards), and encourages you to write your code using these new features. This is a good thing, since you’re not learning a custom syntax that will only work with Aurelia.
Check it out if this sounds interesting to you!
➡️ See the Aurelia JavaScript Framework on GitHub
Pros & cons of Aurelia
Pros
- Powerful and composable
- Forward-thinking
- High extensibility
Cons
- Small community
- Poor documentation
- Steep learning curve
💻 Where to learn it: Aurelia Fundamentals on Pluralsight
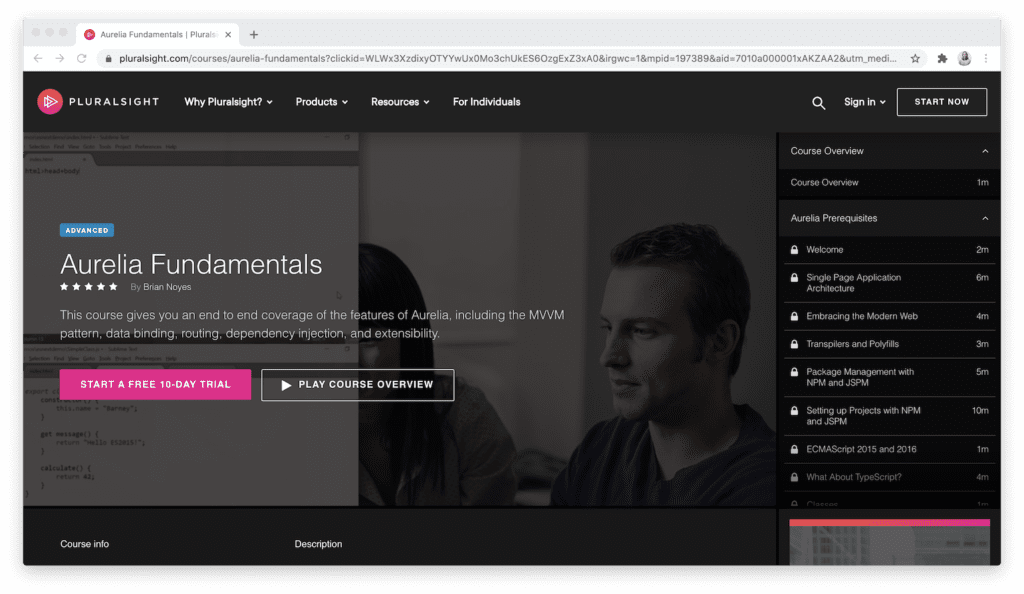
💡 What the course covers: How to leverage all of the key features of Aurelia, including UI composition, two-way data binding, client side routing and navigation, and more.
⬇️ Course facts:
- Course Name: Aurelia Fundamentals
- Platform: Pluralsight
- Instructed by: Brian Noyes
- Price: $29/month with a Pluralsight subscription
📈 Skill level: Intermediate
☝️ Back to the table of contents
Start coding now
Stop waiting and start learning! Get my 10 tips on teaching yourself how to code.
Vue.js: the Progressive JavaScript Framework
Vue.js is an open-source progressive JavaScript framework created initially by Evan You in 2014 while he was working for Google. Since then, Vue.js has changed a lot. It’s been rewritten and refined into a great SPA.
One of Vue’s greatest strengths is its progressiveness. In other words, you can progressively add Vue into your application. Unlike AngularJS and Ember, you can easily mix and match Vue into projects.
Some of its features include components, templates, transitions, and two-way data binding, as well as a focus on reactivity.
Reactivity occurs when you change or update any of Vue’s JavaScript objects. This in turn will update the Vue’s templates. Vue.js uses something called a Shadow DOM that makes rendering the page fast.
I’ve had a lot of time to work on Vue. In fact, I wrote a book on it!
Check out this podcast episode all about Vue!
➡️ See the Vue.js JavaScript Framework on GitHub
Pros & cons of Vue.js
Pros
- Progresssive; you can mix and match
- Fast to download and install; tiny size
- Easy to learn
Cons
- Many elements of the framework are only available in Chinese
- Small community
- Can be hard to debug issues
💻 Where to learn it: Vue – The Complete Guide (w/ Router, Vuex, Composition API) on Udemy
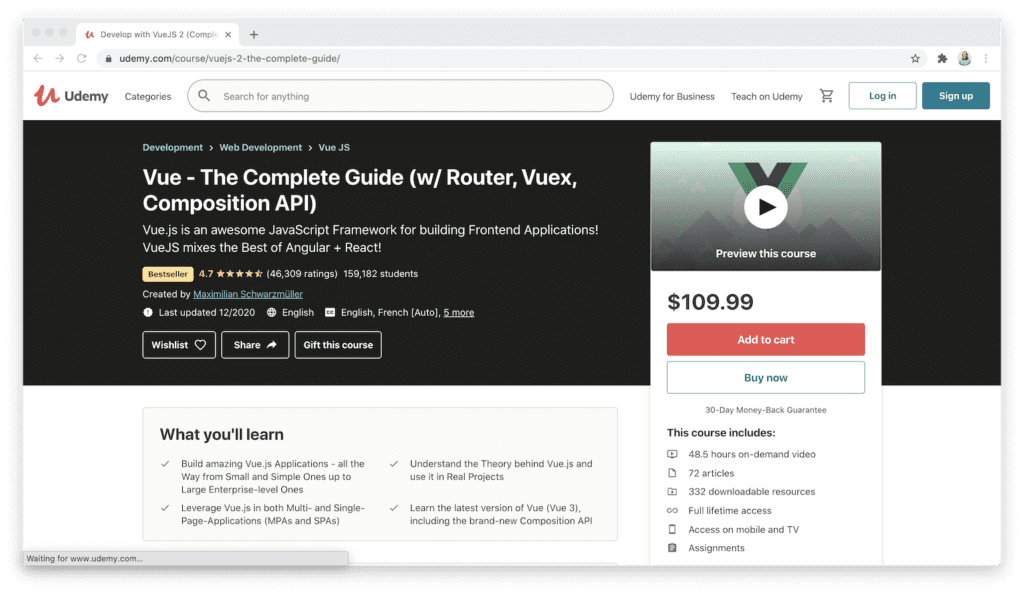
💡 What the course covers: How to build Vue.js applications—all the way from small and simple ones up to large, enterprise-level ones.
⬇️ Course facts:
- Course Name: Vue – The Complete Guide (w/ Router, Vuex, Composition API)
- Platform: Udemy
- Instructed by: Maximilian Schwarzmüller
- Price: $84.99 (but Udemy often has sales)
- 📈 Skill level: Intermediate
☝️ Back to the table of contents
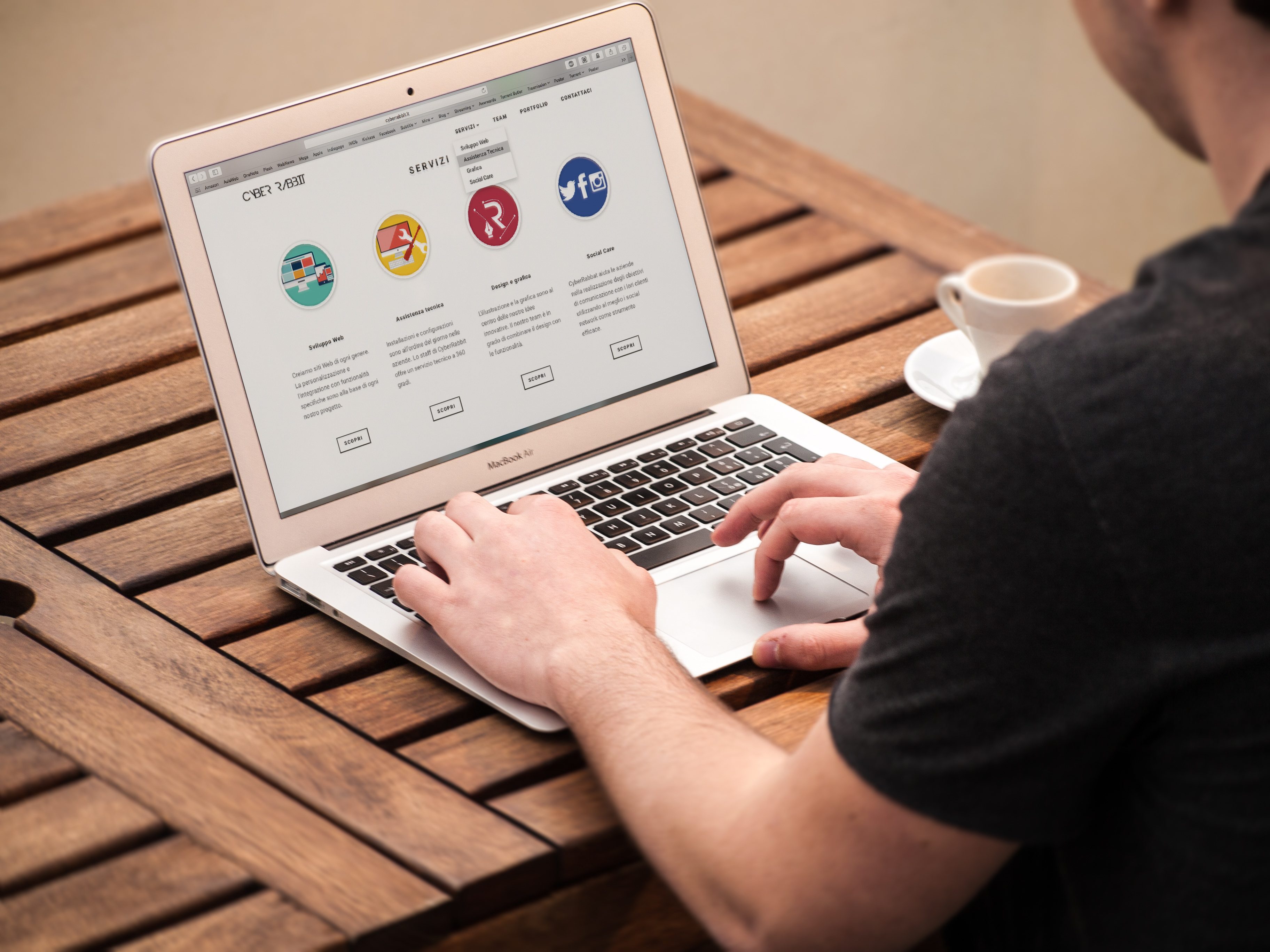
Ready to level up your JavaScript skills even further? Check out Udacity’s Intermediate JavaScript Nanodegree program to learn a more advanced set of JavaScript skills.
TL;DR: the Most Popular JavaScript Libraries and Frameworks
For those of you that are new to web development – you’ll be hearing a lot of cynical people talk about how the JavaScript world has become too fast-paced. They’ll groan that new JavaScript libraries and frameworks are coming out too quickly and that everyone is trying to do the same thing.
They aren’t wrong that it’s fast-paced, but that shouldn’t stop you from going out and learning about these JavaScript frameworks and libraries. I’ve only given you a taste of each one. It’s up to you to pick one up and start learning. For more advice on which to pick, learn about the different JavaScript stacks.
When I mentor new developers, I recommend that they pick a team. After you learn the basics (HTML, JavaScript, and CSS) you have a lot of choices. Don’t try to do everything at once, though: pick one framework and get good at it. You don’t need to learn them all. You just need to learn at least one so you can get your foot in the door somewhere.
Good luck!
About the Author
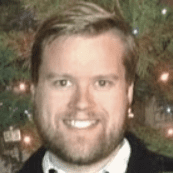
Erik Hanchett is a software developer, YouTuber, blogger, and sushi aficionado. He’s been writing code for over 10 years. Erik’s authored two books, the Ember.js Cookbook and the soon to be released Vue.js in Action. He currently resides in Reno, Nevada, with his wife and two kids. You can find more information from Erik at his blog www.programwitherik.com.
Note: there are affiliate links in this post.